Code Example Guidelines
Note
This page provides general guidance for creating code examples in our docs, irrespective of language. Language-specific style guidelines and coding standards are in progress.
Code examples demonstrate how to use our products programmatically. High-quality, well-maintained code improves our docs' usability, builds our credibility with users, and helps reduce reported issues.
What is a Code Example?
A code example is a block of code of any size that is set apart from regular text through a code directive. For details on valid block-level code directives, see the Code Examples reference.
Use block-level directives for all code examples. A code block is visually and functionally distinct from other page elements. It defines and displays its content as code, instead of regular text. It is also easier to read and better interpreted by robot users (such as screen readers, AI models, and crawlers).
Do not use inline-level markup for code examples. Use inline code markup to format code-related references within text in
monospace
(such as method names).Inline code text
Code block
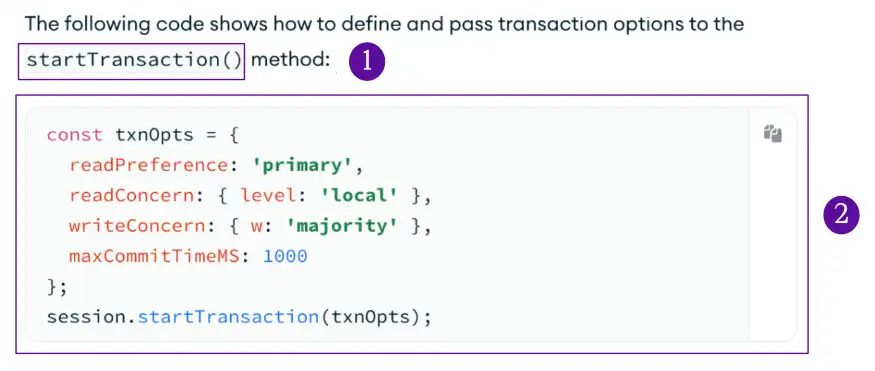
Types of Code Examples
Note
Although "code example" and "snippet" are often used interchangeably, this guidance uses "snippet" to mean a context-less block of code.
We categorize code examples into the following types:
Usage Examples: Standalone code blocks that show how to perform a task, including the relevant setup and context.
Snippets: Code that illustrates a specific concept or detail in the context of a larger example, tutorial, or reference page.
Sample Applications: Runnable applications demonstrating broader use cases.
Usage Examples
Usage examples are self-contained, actionable code blocks that show how to accomplish a specific task using MongoDB tools, drivers, or APIs. Usage examples include enough information to understand, modify, and run the code contained in the code block (for example, a single code block that contains all declared variables and includes comments to indicate which placeholders to update).
using MongoDB.Driver; // Replace the following with your MongoDB connection string const string connectionUri = "mongodb://<db_username>:<db_password>@<hostname>:<port>/?connectTimeoutMS=10000"; var client = new MongoClient(connectionUri);
Snippets
Snippets are narrowly scoped code blocks that help explain a specific concept or detail. They are typically used as part of a broader explanation or tutorial, and are often meaningful only within that context.
Snippets are intended to provide information. They aren't required to be valid or runnable code. In some cases, snippets may contain intentionally incomplete or incorrect code for demonstration purposes (for example, a snippet showing all possible arguments for a command).
Snippets fall into one of the following subtypes:
Non-MongoDB command: a command-line (CLI) command for any non-MongoDB tooling (for example,
mkdir
,cd
, ornpm
), often used in the context of a tutorial.dotnet run MyCompany.RAG.csproj Syntax example: an example of the syntax or structure for an API method, an Atlas CLI command, a
mongosh
command, or other MongoDB tooling.mongodb+srv://<db_username>:<db_password>@<clusterName>.<hostname>.mongodb.net Return example: an example of an object, such as a JSON blob or sample document, returned after executing a corresponding piece of code. Commonly included as the output of an
io-code-block
.A timeout occurred after 30000ms selecting a server using ... Client view of cluster state is { ClusterId : "1", State : "Disconnected", Servers : [{ ServerId: "{ ClusterId : 1, EndPoint : "localhost:27017" }", EndPoint: "localhost:27017", State: "Disconnected" }] } Configuration object example: an example configuration object, often represented in YAML or JSON, enumerating parameters and their types.
apiVersion: atlas.mongodb.com/v1 kind: AtlasDeployment metadata: name: my-atlas-cluster spec: backupRef: name: atlas-default-backupschedule namespace: mongodb-atlas-system
Sample Applications
Sample applications are complete, runnable programs that connect multiple discrete pieces of code. Sample apps may include error handling, framework integrations, or frontend UI elements.
General Guidelines
Our code examples should always follow generally accepted coding and security best practices, and all other applicable guidelines in this Style Guide that don't conflict with language-specific standards. Remember that users copy and use these code examples outside of our docs.
Keep the following in mind as you write code examples:
Treat code like writing: Keep it simple, readable, and relevant to the task.
Write code that is easy to understand, even if it isn't the most efficient or clever.
Introduce each code block with context, as you would a list or table.
Include any prerequisites or code dependencies needed for a piece of code.
Use descriptive names that clearly convey the purpose of the code element (e.g. variable, function, class) or placeholder.
If a code is not intended to be directly used or adapted, such as a return object example snippet, make sure the code block is not copyable. To learn how to set the copyable option, see Code Examples.
Don't write code examples for anti-patterns. If you need to note an anti-pattern or a commonly made mistake, use the surrounding text, an admonition, or a code comment, and ensure that it cannot be mistaken for a recommended pattern.
If a code example is not production-worthy in a significant way, communicate this to readers through code comments and the surrounding text.
Never use real customer data or hard code secrets in your code. If you're unsure how to handle secrets in your code, reach out to the DevDocs team.
Use code comments to explain or call out important details, including:
Non-obvious logic or intent. Don't restate the code.
Omitted or truncated code sections. If you need to omit code, use a comment to indicate what is missing and why.
Test every code example to ensure it works as intended:
When writing or reviewing, run the code as it displays on the page.
For tutorials or multi-step examples, begin at the starting point and complete all steps exactly, including any prerequisites or setup. Don't skip steps or assume they're correct.
If you have to make changes or take additional steps to get an example to work, make sure those details are reflected in the documentation.
Note
For any questions or for help writing or testing code examples, reach
out to the @DevDocs
team or use the #ask-devdocs
Slack channel.
Considerations for LLMs
Unlike human readers, the robots that consume our docs (such as LLMs and other AI models) can't infer meaning or intent from context. Robot users struggle with ambiguity, unclear boundaries, partial or implicit information, and non-standard patterns.
To ensure that our code examples are robot friendly, keep the following in mind:
Prefer atomic code examples. Code should be as self-contained and self-descriptive as possible so that robot users can understand the code more easily.
Explain intent, purpose, or expected code behavior through surrounding text and code comments.
Don't interrupt a single code block with text explanations. Keep explanatory text before or after the code block, and use code comments for explanations within the code block.
Prefer conventional file structure, names, and canonical patterns (for example,
main()
,index.js
,connect()
). Avoid unnecessary aliasing or non-standard structures unless explained clearly.Use consistent, descriptive names that indicate purpose. Avoid vague names like
foo
,x
, ordoStuff()
.Specify the code language for every code block, including output examples in an
io-code-block
. For a complete list of supported languages, see the leafygreen-ui GitHub repository. If you are unsure which language to use or need a new language added to the list, reach out to the DevDocs team.Clearly mark and explain any placeholders, omissions, or truncated code through code comments.